Javascript Blackjack Github
/*Object Oriented Blackjack |
A Game has: Players |
Dealer |
Deck |
A Player / Dealer has: Score |
Cards |
A Score has: Game Logic |
Current Score |
A Deck has: Cards |
*/ |
function Game() { |
this.currentTurnIndex = 0; |
this.deck = new Deck(); |
} |
function Deck() { |
this.cards = []; |
this.cardsDrawn = 0; |
var suits = ['spades', 'diamonds', 'hearts', 'clubs']; |
var names = ['ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'jack', 'queen', 'king']; |
for (var suit in suits) { |
for (var name in names) { |
this.cards.push(new Card(names[name], suits[suit])); |
} |
} |
} |
Deck.prototype.getCard = function () { |
if (this.cards.length this.cardsDrawn) { |
return null; |
} //case: check if all cards drawn |
var random = Math.floor(Math.random() * (this.cards.length - this.cardsDrawn)); |
var temp = this.cards[random]; |
//swap chosen card with card last in array |
this.cards[random] = this.cards[this.cards.length - this.cardsDrawn - 1]; |
this.cards[this.cards.length - this.cardsDrawn - 1] = temp; |
this.cardsDrawn++; |
return temp; |
}; |
function Card(name, suit) { |
this.name = name; |
this.suit = suit; |
} |
Card.prototype.image = function () { |
return 'http://www.jonarnaldo.com/sandbox/deck_images/' + name + '_of_' + suit + '.png'; |
}; |
Card.prototype.value = function () { |
if (this.name 'jack' 'queen' 'king') { |
return [10]; |
} else if (this.name 'ace') { |
return [1, 11]; |
} else { |
return parseInt(this.name, 10); |
} |
}; |
function Player() { |
//this.name; |
this.cards = []; |
} |
Player.prototype.addCard = function () { |
this.cards.push(deck.getCard()); |
}; |
Player.prototype.score = function () { |
var score = 0; |
var aces = []; |
for (var i = 0; i < this.cards.length; i++) { |
var value = this.cards[i].value() // value array ex.[10] |
if (value.length 1) { |
score += value[0]; |
} else { |
aces.push(value); |
} |
} |
for (var j = 0; j < aces.length; j++) { |
if (score + aces[j].value[1] <= 21) { |
score + aces[j].value[1]; |
} else { |
score + aces[j].value[0]; |
} |
} |
return score; |
}; |
var deck = new Deck(); |
var player1 = new Player(); |
$('#getCard').click(function () { |
player1.addCard(); |
var cardName = player1.cards[player1.cards.length-1].name; |
var cardSuit = player1.cards[player1.cards.length-1].suit; |
$('#table').append(cardName + cardSuit); |
}); |

Bet this round: 0. Hit Hold Restart Rules. A simple blackjack game in JavaScript. Contribute to stayko/blackjack-js development by creating an account on GitHub. So naturally, with blackjack, I just ignored the suites, since they don’t matter. Then, I went through and changed all the KINGS, QUEENS and JACKS value to 10. Finally for the Ace, my thought on that is that, you always want the value of the Ace to be 11, unless your total hand value is over 21, then you’d want the Ace to be 1, since you. HTML/Javascript blackjack game. JavaScript - Last pushed Jul 7, 2015 - 0 stars apkiernan/apkiernan.github.io. Personal/Test site JavaScript. (In Progress) A blackjack game, created to practice javascript fundamentals, including functions, objects and loops. Displays a list of random exercises with a countdown clock. User can select the number of exercises and duration.
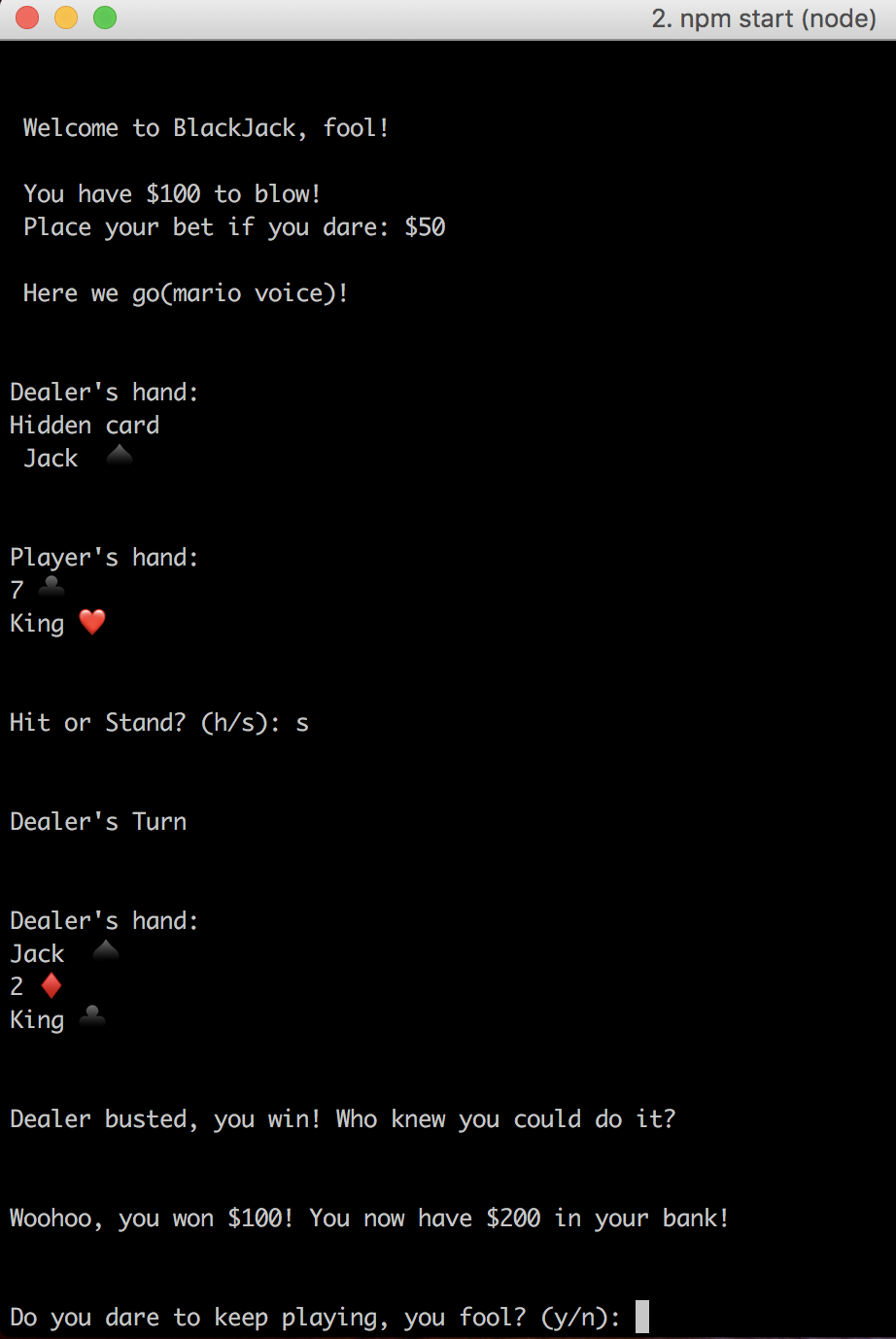
Javascript Blackjack Github Interview
commented Jun 13, 2017
Blackjack Javascript Code
My coding bootcamp is making me code a Blackjack Hand Calculator. I have to turn it in soon. I'm failing. JavaScipt is fking me. |